Custom RXJS Operator : delayFirstEmmisionBy
Oct 14, 2020 11:22 · 33 words · 1 minute read
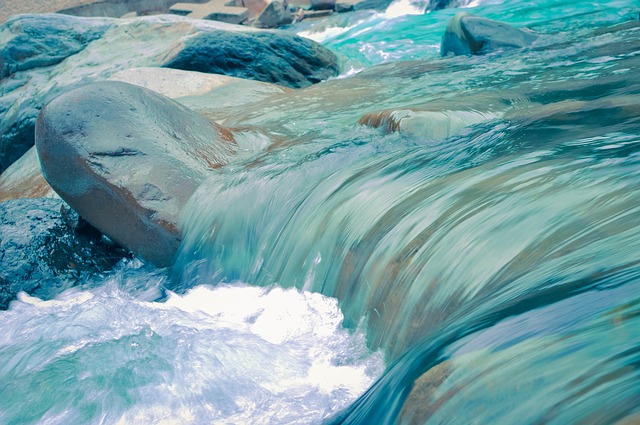
I ran accross an use case when first emmision needed to be delayed by X seconds and therefore had to resort to making my own custom operator.
I ran accross an use case when first emmision needed to be delayed by X seconds and therefore had to resort to making my own custom operator.